Prerequisite
Download Kibana and ElasticSearch on Windows
Extract zip files of ElasticSearch and Kibana
Under CMD
Browse bin folder under ElasticSearch Folder
Type elasticsearch
You should be able to access Elastic Search at "http://localhost:9200"
Under CMD
Browse bin folder under Kibana Folder
Type kibana
You should be able to access Kibana at "http://localhost:5601"
Now We need to Setup Selenium to push the Test Execution Status to ElasticSearch
We will create TestStatus class (This will provide Sample Json which contains information about test Execution, this will be pushed to Elastic Search)
package com.selenium.grid;
import com.fasterxml.jackson.annotation.JsonProperty;
public class TestStatus {
@JsonProperty("testClass")
private String testClass;
@JsonProperty("description")
private String description;
@JsonProperty("status")
private String status;
@JsonProperty("executionTime")
private String executionTime;
public void setDescription(String description) {
this.description = description;
}
public void setExecutionDate(String executionTime) {
this.executionTime = executionTime;
}
public void setStatus(String status) {
this.status = status;
}
public void setTestClass(String testClass) {
this.testClass = testClass;
}
}
We will create ExecutionListener Class
This class will help in creating TestStatus Json as we can Extract the execution Status of Test cases using TestNg Listener
package com.selenium.grid;
import java.time.LocalDateTime;
import org.testng.ITestContext;
import org.testng.ITestListener;
import org.testng.ITestResult;
public class ExecutionListener implements ITestListener {
private TestStatus testStatus;
public void onTestStart(ITestResult iTestResult) {
this.testStatus = new TestStatus();
}
public void onTestSuccess(ITestResult iTestResult) {
this.sendStatus(iTestResult,"PASS");
}
public void onTestFailure(ITestResult iTestResult) {
this.sendStatus(iTestResult,"FAIL");
}
public void onTestSkipped(ITestResult iTestResult) {
this.sendStatus(iTestResult,"SKIPPED");
}
public void onTestFailedButWithinSuccessPercentage(ITestResult iTestResult) {
//skip
}
public void onStart(ITestContext iTestContext) {
//skip
}
public void onFinish(ITestContext iTestContext) {
//skip
}
private void sendStatus(ITestResult iTestResult, String status){
this.testStatus.setTestClass(iTestResult.getTestClass().getName());
this.testStatus.setDescription(iTestResult.getMethod().getDescription());
this.testStatus.setStatus(status);
this.testStatus.setExecutionDate(LocalDateTime.now().toString());
ResultSender.send(this.testStatus); // This is Next Class that will send Execution Results to //ElasticSearch
}
}
Now we will Create ResultSender Class that will help us in Sending Test Execution Status to Elastic Search
package com.selenium.grid;
import io.restassured.RestAssured;
import java.io.IOException;
import org.apache.http.HttpEntity;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.testng.annotations.Test;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
public class ResultSender {
private static final ObjectMapper OM = new ObjectMapper();
private static final String CONTENT_TYPE = "Content-Type";
private static final String CONTENT_TYPE_VALUE = "application/json";
private static final String ELASTICSEARCH_URL = "http://localhost:9200/app/suite"; // //Note here app is index(we can choose whatever, here it is by the name "app")
@Test
public static void send(final TestStatus testStatus){
RestAssured.baseURI = "http://localhost:9200/app/suite";
System.out.println("In Result Sender");
CloseableHttpClient httpclient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(ELASTICSEARCH_URL);//We will post data in ElasticSea
String JSON_STRING = null;
try {
JSON_STRING = OM.writeValueAsString(testStatus); // writing test status as Json, //using Jackson Object Mapper(OM)
System.out.println("JSON_STRING-->"+JSON_STRING);
} catch (JsonProcessingException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
HttpEntity stringEntity = new StringEntity(JSON_STRING,ContentType.APPLICATION_JSON);
httpPost.setEntity(stringEntity);
CloseableHttpResponse response2=null;
try {
response2= httpclient.execute(httpPost);// executing post method
} catch (ClientProtocolException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
System.out.println("Status-->"+response2.getStatusLine());
}
}
We need Jackson as dependency
6.) Provide the information for Filters and Buckets
7.) See the Test Execution under Visualize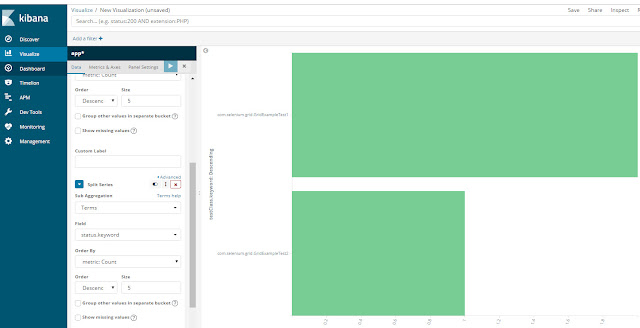
8.) Under Dashboard , you can import all these Visualize
Download Kibana and ElasticSearch on Windows
Extract zip files of ElasticSearch and Kibana
Under CMD
Browse bin folder under ElasticSearch Folder
Type elasticsearch
You should be able to access Elastic Search at "http://localhost:9200"
Under CMD
Type kibana
You should be able to access Kibana at "http://localhost:5601"
Now We need to Setup Selenium to push the Test Execution Status to ElasticSearch
We will create TestStatus class (This will provide Sample Json which contains information about test Execution, this will be pushed to Elastic Search)
package com.selenium.grid;
import com.fasterxml.jackson.annotation.JsonProperty;
public class TestStatus {
@JsonProperty("testClass")
private String testClass;
@JsonProperty("description")
private String description;
@JsonProperty("status")
private String status;
@JsonProperty("executionTime")
private String executionTime;
public void setDescription(String description) {
this.description = description;
}
public void setExecutionDate(String executionTime) {
this.executionTime = executionTime;
}
public void setStatus(String status) {
this.status = status;
}
public void setTestClass(String testClass) {
this.testClass = testClass;
}
}
We will create ExecutionListener Class
This class will help in creating TestStatus Json as we can Extract the execution Status of Test cases using TestNg Listener
package com.selenium.grid;
import java.time.LocalDateTime;
import org.testng.ITestContext;
import org.testng.ITestListener;
import org.testng.ITestResult;
public class ExecutionListener implements ITestListener {
private TestStatus testStatus;
public void onTestStart(ITestResult iTestResult) {
this.testStatus = new TestStatus();
}
public void onTestSuccess(ITestResult iTestResult) {
this.sendStatus(iTestResult,"PASS");
}
public void onTestFailure(ITestResult iTestResult) {
this.sendStatus(iTestResult,"FAIL");
}
public void onTestSkipped(ITestResult iTestResult) {
this.sendStatus(iTestResult,"SKIPPED");
}
public void onTestFailedButWithinSuccessPercentage(ITestResult iTestResult) {
//skip
}
public void onStart(ITestContext iTestContext) {
//skip
}
public void onFinish(ITestContext iTestContext) {
//skip
}
private void sendStatus(ITestResult iTestResult, String status){
this.testStatus.setTestClass(iTestResult.getTestClass().getName());
this.testStatus.setDescription(iTestResult.getMethod().getDescription());
this.testStatus.setStatus(status);
this.testStatus.setExecutionDate(LocalDateTime.now().toString());
ResultSender.send(this.testStatus); // This is Next Class that will send Execution Results to //ElasticSearch
}
}
Now we will Create ResultSender Class that will help us in Sending Test Execution Status to Elastic Search
package com.selenium.grid;
import io.restassured.RestAssured;
import java.io.IOException;
import org.apache.http.HttpEntity;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.testng.annotations.Test;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
public class ResultSender {
private static final ObjectMapper OM = new ObjectMapper();
private static final String CONTENT_TYPE = "Content-Type";
private static final String CONTENT_TYPE_VALUE = "application/json";
private static final String ELASTICSEARCH_URL = "http://localhost:9200/app/suite"; // //Note here app is index(we can choose whatever, here it is by the name "app")
@Test
public static void send(final TestStatus testStatus){
RestAssured.baseURI = "http://localhost:9200/app/suite";
System.out.println("In Result Sender");
CloseableHttpClient httpclient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(ELASTICSEARCH_URL);//We will post data in ElasticSea
String JSON_STRING = null;
try {
JSON_STRING = OM.writeValueAsString(testStatus); // writing test status as Json, //using Jackson Object Mapper(OM)
System.out.println("JSON_STRING-->"+JSON_STRING);
} catch (JsonProcessingException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
HttpEntity stringEntity = new StringEntity(JSON_STRING,ContentType.APPLICATION_JSON);
httpPost.setEntity(stringEntity);
CloseableHttpResponse response2=null;
try {
response2= httpclient.execute(httpPost);// executing post method
} catch (ClientProtocolException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
System.out.println("Status-->"+response2.getStatusLine());
}
}
We need Jackson as dependency
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.9.4</version>
</dependency>
Now Last Step is to Use the Listener for Test Execution
For Every Test Class we need to add this listener
@Listeners(ExecutionListener.class)
public class GridExampleTest1 {
First We need to Execute the Test Script so that when Data is pushed to http://localhost:9200/app/suite
Index "app" has data in Elastic, so that Kibana can search in that index
1.) Create Index Pattern ("app") in Kibana , Click on Next
2.)Click on Create Index Pattern
3.)Under Dashboard ,You will see all test cases executed
4.) Visualize, Create Visualization accordingly
5.) Visualize
6.) Provide the information for Filters and Buckets
7.) See the Test Execution under Visualize
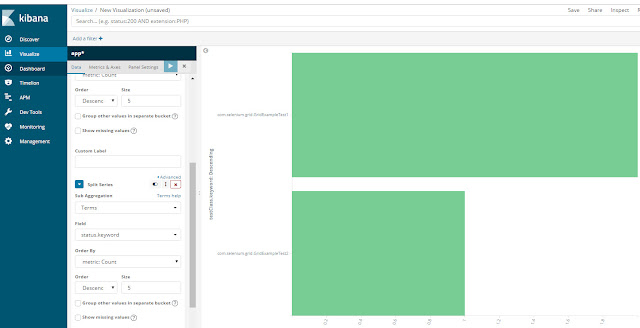
8.) Under Dashboard , you can import all these Visualize
No comments:
Post a Comment